What is DOM in Javascript?
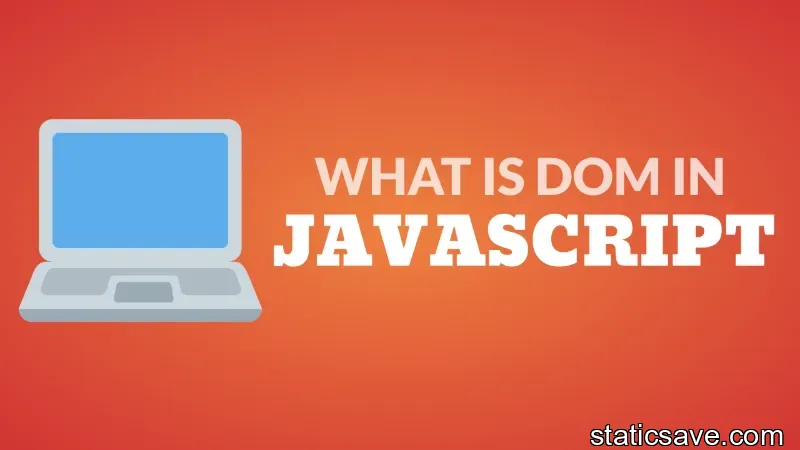
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a document as a tree-like structure, with each node in the tree representing a different aspect of the document, such as an element, an attribute, or text content. The DOM is language and platform-independent, which means it can be used by any programming language, such as JavaScript, Python, C# etc.
In JavaScript, the DOM is used to represent an HTML or XML document as a tree-like structure of objects in memory. Each object in the tree corresponds to a different aspect of the document, such as an element, an attribute, or text content. The objects in the DOM tree have properties and methods that can be used to access and manipulate the contents of the document.
When a web page is loaded, the browser parses the HTML or XML code and builds a corresponding tree of objects in memory, which can be accessed and manipulated using JavaScript. This makes it possible to create dynamic and interactive web pages that can update in response to user actions or other events.
The DOM is typically represented as a tree, where the top-most element is called the "root" and all other elements branch out from it. Each element in the tree can have a parent, children and siblings.
Also Read: Learning Javascript for Web Development
How does DOM work in JavaScript?
The DOM works by representing an HTML or XML document as a tree-like structure, with each node in the tree representing a different aspect of the document, such as an element, an attribute, or text content. When a web page is loaded, the browser parses the HTML or XML code and builds a corresponding tree of objects in memory, which can be accessed and manipulated using JavaScript.
JavaScript can interact with the DOM using a variety of methods and properties, such as:
- document.getElementById() - Returns a reference to the element with a specific id attribute
- document.getElementsByTagName() - Returns a collection of elements with a specific tag name
- element.innerHTML - Gets or sets the HTML content within an element
- element.style - Gets or sets the inline style of an element
For example, you can use JavaScript to change the content of a specific element in the DOM by finding a reference to the element and then setting the element's innerHTML property to a new value, or you can change the style of an element by accessing the style property and setting it to new values.
JavaScript can also respond to events such as user input or page loading by using Event Listeners, which can be added to a particular element of the DOM, and when the specific event is triggered, the corresponding function will be executed.
In general, DOM provides a way for JavaScript to access and manipulate the contents of a web page in a way that is independent of the underlying HTML or XML code, allowing developers to create dynamic, interactive web pages that can update in response to user actions or other events. It also makes it possible to create cross-browser compatible code, it improves the performance, it allows for event-driven programming, and it is extensible.
What are the benefits of using DOM in JavaScript?
There are several benefits to using the Document Object Model (DOM) in JavaScript:
Separation of concerns: The DOM allows for a separation of concerns between the structure of the document (HTML or XML) and the behavior (JavaScript). This makes it easier to maintain and update the code, as changes to the structure of the document do not affect the JavaScript code, and vice versa.
Dynamic updates: The DOM provides a way for JavaScript to programmatically access and manipulate the contents of a document, allowing for dynamic updates to the web page in response to user actions or other events. This makes it possible to create interactive and dynamic web pages without the need for a page refresh.
Consistent API: The DOM provides a consistent API across all browsers, making it easier to write cross-browser compatible code. This ensures that the same code can be used to manipulate the contents of a web page regardless of the browser being used to view it.
Better performance: DOM structure can be cached in memory and accessed quickly compared to parsing the entire HTML again and again, resulting in better performance.
Event-driven: DOM can be used to create event-driven applications. JavaScript can respond to events such as user input or page loading by using Event Listeners, which can be added to a particular element of the DOM, allowing for a more interactive and responsive user experience.
Extensibility: DOM is designed to be extensible, allowing for the creation of new elements and attributes and the addition of custom functionality. This allows for the creation of new and innovative features that can be incorporated into web pages.
Also Read: Why JavaScript is Important for Web Developers?
Conclusion
In conclusion, the Document Object Model (DOM) is an essential part of JavaScript programming. It provides a programming interface for HTML and XML documents, representing the structure of a document as a tree of objects, which can be accessed and manipulated using JavaScript. The DOM allows for the separation of concerns between the structure of the document and the behavior, enabling dynamic updates to the web page in response to user actions or other events. It also provides a consistent API across all browsers and a way to cache in memory for better performance. Additionally, it enables Event-driven programming and Extensibility. With its powerful capabilities, the DOM plays a critical role in creating dynamic, interactive and cross-browser compatible web pages.